Corrected HTML Code:
Welcome, fellow Unity developers! Today, we delve into the art of implementing keyboard controls in your Unity projects. This guide is designed to empower you with practical insights, backed by research and real-world examples.
Why Keyboard Controls Matter
Keyboard controls are essential for creating intuitive, responsive games. They offer a familiar interface that users can easily grasp, enhancing the overall gaming experience. As a Unity developer, mastering keyboard control implementation is a crucial step towards crafting engaging and immersive games.
Getting Started: Understanding Input Manager
The first step in implementing keyboard controls is understanding Unity’s Input Manager. This powerful tool allows you to map keys to specific functions, making your game responsive and user-friendly.
Creating Keyboard Control Schemes
To create a keyboard control scheme, navigate to Edit > Project Settings > Input Manager. Here, you can define keybindings for various actions in your game. For instance, you might assign the ‘W’ key to move forward or the ‘Spacebar’ to jump.
Real-life Example: A Simple Platformer
Let’s consider a simple platformer game as an example. We’ll map the ‘W’ and ‘A/D’ keys for movement, and the ‘Spacebar’ for jumping. Here’s how you might set this up in the Input Manager:
<p> Horizontal Axis: Horizontal
- On Axis Down: Horizontal -1
- On Axis Up: Horizontal 1
Jump Button: Space</code></pre>
Implementing Keyboard Controls in Scripts
Once you've defined your keybindings, it's time to implement them in your scripts. Here's a simple example of how to move a game object based on the Horizontal axis input:
<pre><code>void Update()
{
float horizontal = Input.GetAxis("Horizontal");
transform.Translate(Vector3.right * horizontal * Time.deltaTime);
}</code></pre>
Tips and Tricks
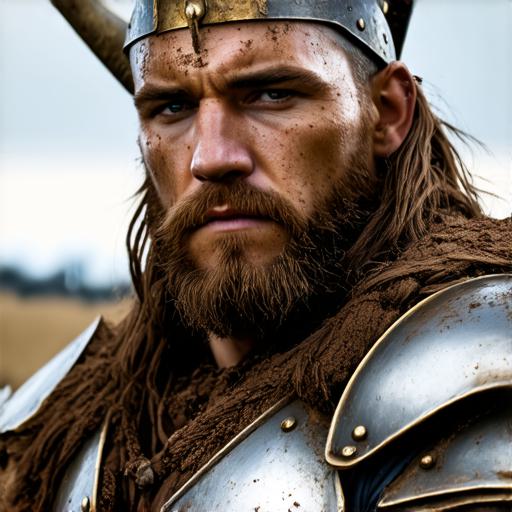
Use the `Input.GetKeyDown()` function to trigger events when a key is pressed, such as jumping or firing a weapon.
Consider using `Input.GetAxisRaw()` for more precise control over input. This function returns an integer instead of a float, making it easier to handle discrete inputs like button presses.
Don't forget to test your keyboard controls thoroughly to ensure a smooth gaming experience.
FAQs
1. Why should I use keyboard controls in my Unity game?
Keyboard controls offer a familiar, intuitive interface that users can easily grasp. They are essential for creating responsive and engaging games.
2. How do I create a new keyboard control scheme in Unity?
Navigate to Edit > Project Settings > Input Manager, then define keybindings for various actions in your game.
3. What is the difference between Input.GetAxis() and Input.GetAxisRaw()?
`Input.GetAxis()` returns a float value, while `Input.GetAxisRaw()` returns an integer value, making it easier to handle discrete inputs like button presses.